Streamlining Route Data Binding with Angular Signal Inputs
Reading time
~ 3 min read
Tags
angular
Author
Mustapha Aouas
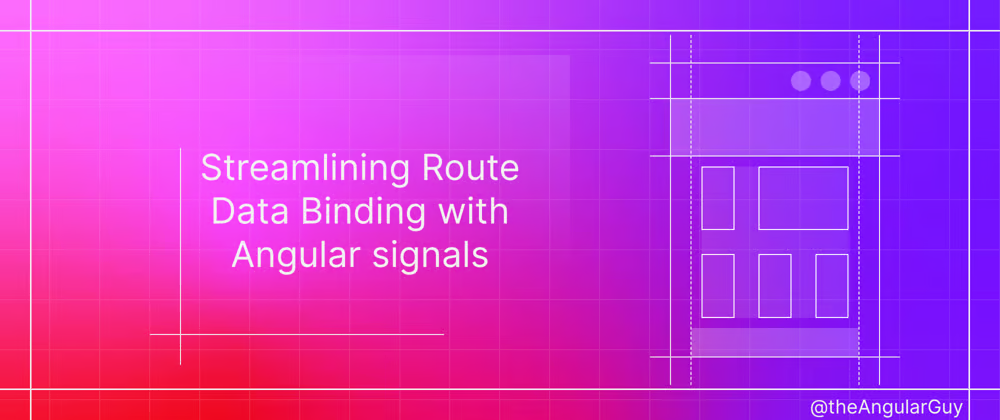
Angular's latest versions introduce a powerful combination of signal inputs, and route binding, simplifying how we handle route information in components. This article will explore this relatively new feature and how it integrates with Angular's reactive programming model.
StackBlitz example: Angular route binding with signals.
How Route Data Binding Works with Signals
In the latest versions of Angular, we can bind route information directly to component signal inputs, making our code more reactive and easier to manage.
Let's start with a simple route configuration:
export const DASHBOARD_ROUTES: Routes = [
{
path: 'dashboard',
component: DashboardComponent,
},
];
Now, instead of using @Input()
decorators or injecting ActivatedRoute
,
we can use signal inputs in our component:
import { Component, input } from '@angular/core';
@Component({
selector: 'app-dashboard',
template: `
<h1>Selected tab: {{ tab() }}</h1>
<!-- Rest of the template -->
`
})
export class DashboardComponent {
readonly tab = input<string>();
// The router will automatically update this signal
// Example URL: http://localhost:4200/dashboard?tab=Angular
}
For more complex scenarios, including path parameters, route data, and resolved data:
export const DASHBOARD_ROUTES: Routes = [
{
path: "dashboard/:id",
component: DashboardComponent,
data: { title: "Dashboard title" },
resolve: { dashboardData: DashboardDataResolver }
},
];
@Component({
// ... component metadata
})
export class DashboardComponent {
readonly id = input<string>();
readonly title = input<string>();
readonly dashboardData = input<SomeType>();
// These signals will be automatically updated by the router
}
Data Binding Precedence and Input Aliasing
Data Binding Precedence
When route data has the same name across different sources (resolved data, route data, path params, query params), the binding follows this order:
- Resolve Data
- Route data
- Path params
- Query params
To avoid ambiguity, you should use different names.
Input Aliasing
Consider this snippet:
readonly dashboardId = input('', {alias: 'id'});
In the code above, for the route /dashboard/:id
, we can use dashboardId
in
the component while still matching the :id
parameter of the route.
This can improve maintainability as a change in to an external naming (route param) doesn't require many changes to internal component code. Also, it can be more readable depending on the context, as variables within the component have clear, descriptive names.
Steps To Try It Out
Enable It In Your Application's Router Configuration
For standalone applications:
bootstrapApplication(App, {
providers: [
provideRouter(routes,
withComponentInputBinding()
)
],
});
For NgModules:
@NgModule({
imports: [
RouterModule.forRoot([], {
bindToComponentInputs: true
})
],
})
export class AppModule {}
Migrate To The New API
- Remove
ActivatedRoute
service injections. - Replace
@Input()
properties with input signals. - Enable
bindToComponentInputs
orwithComponentInputBinding
in your router configuration as we saw in the section above.
Example migrating from this:
@Component({/* ... */})
export class MyComponent implements OnInit {
private route = inject(ActivatedRoute);
id$ = this.route.params.pipe(map(params => params['id']));
ngOnInit() {
this.id$.subscribe(id => { /* do something */ });
}
}
To this:
@Component({/* ... */})
export class MyComponent {
readonly id = input<string>();
constructor() {
effect(() => { /* do something with this.id() */ });
}
}
Note: In the next angular version (19), there will be a new way to share data using
routerOutletData
directly in the<router-outlet />
. We will cover this in the next article.
Wrapping Up
The combination of signal inputs and route binding in Angular represents a significant step forward in simplifying how we handle route information in our applications.
This approach offers several benefits:
- Reduced boilerplate code: We no longer need to inject the
ActivatedRoute
service or set up (some-what complex) RxJS pipelines to handle route parameters. The router automatically updates our signals. - Improved readability: By using signals, our component code becomes more declarative and easier to understand (at a glance).
- Simplified testing: It's straightforward to test components using signal inputs
for route binding compared to
ActivatedRoute
+ observables. - Future-proofing: As Angular continues to evolve, signals are likely to become more central to the framework. Adopting them can help future-proof our applications.
While there are some considerations to keep in mind, such as the priority of route information binding and the need for clear naming conventions (maybe using input aliases), the benefits of using signal inputs with route binding far outweigh these minor caveats in my opinion.